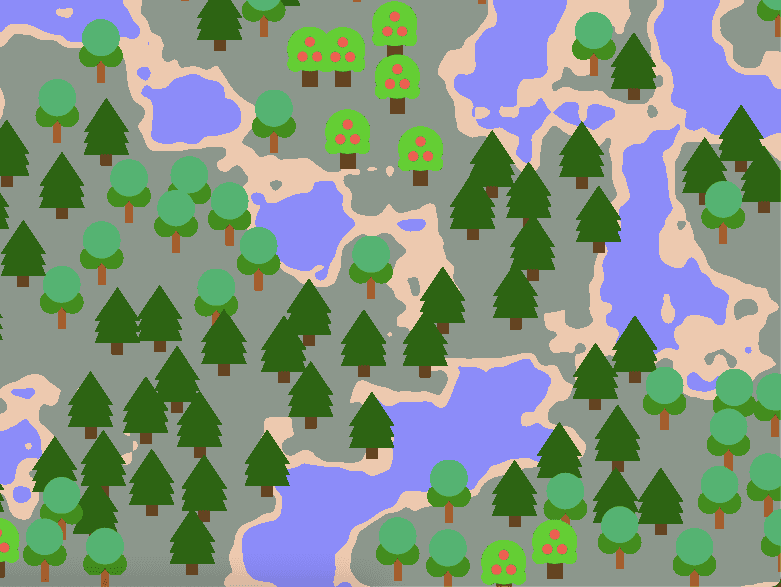
Coding Challenge: Procedurally Generated Forest Terrain
Published: Jan 27, 2025
In this coding challenge, I learned how to make procedurally generated forest landscapes with Javascript and Canvas. Forests are generated by using the Perlin Noise and Poisson Disk Sampling algorithms. This project first renders some generated terrain of grass, dirt, and water by using the Perlin Noise algorithm. Then trees are added to the grassy areas using the Poisson Disk Sampling algorithm. The user can play with various settings and generate new forests. Every forest that is generated will be unique due to the nature of the Perlin Noise and Poisson Disk Sampling algorithms, which produce a sort of organized randomness.
You can interact with the project full screen on Codepen: https://codepen.io/fleemaja/full/XJWJXge. Use the menu to play with settings and create new forests. You can also see the project here:
Perlin Noise is a type of gradient noise developed by computer scientist Ken Perlin in 1983. I like to think of it as a sort of smooth randomness, which can create textures that seem organic and life-like. Values are not just completely random, they are related to neighboring values. This can produce computer graphics that look like realistic clouds with gradients of dark and light, or a map of terrain like I’ve made for this project. Here is a video of Youtuber RachelfTech showing how to make procedural terrain with Perlin Noise:
In the video, it is shown how to make procedural 2D terrain, like I've made for my project. To make the illusion of a terrain map, you set the pixels of the background to colors based on a 2D perlin noise value that is calculated using both an x and y parameter as it moves down and across the screen. Perlin noise outputs a value between 0 and 1. Values are not completely random, they are related to neighboring values, so it produces a patchy randomness, which is perfect for generating this terrain map with grass, dirt, and water. To draw these different colors to the screen you just set pixel's color within a certain perlin noise value to green for the grass (e.g. 0 - 0.33), another range to brown for the dirt (e.g. 0.33 - 0.66), and the rest to blue for the water.
Next, I populated the terrain with some trees. I did this by using the Poisson Disk Sampling algorithm. This algorithm allow you to cover an area with randomized points that are separated by a specified minimum distance, resulting in a natural looking pattern that is organized but still uniquely random. To run this algorithm you give it a starting point and then it will choose random vectors a certain distance away from the point as new and "active" points. From these active points it looks for potential new spots by testing random vectors a certain distance away from each current point. If they find a point that is a minimum distance away from all of the other points in the area, that point is then added as a new and active point. The algorithm completes when each point becomes inactive after a certain number of failed attempts at finding a new point (whether it is too close to other points or the boundaries of the specified area).
You can learn about the Poisson Disk Sampling algorithm in more detail in this video by The Coding Train:
One of the tricky things I had to figure out was how to avoid adding and drawing trees in the water areas. To do this, I created an array of all of the Perlin Noise values for every terrain point (a massive array for every pixel in the canvas window). I then checked all samples in the Poisson Disk Sampling algorithm using their x and y positions in the terrain grid to find the terrain grid’s noise value at that position. If it was in the range of values I assigned as “water”, I did not add a tree object to draw to the screen at that position. It still runs the Poisson Disk algorithm as normal though, adding unseen points to the poisson disk grid, which spans the whole window area and terrain grid.
Another detail I added was clustering the types of trees together using Perlin Noise. This works like the terrain. It creates bunches of the same kind of trees together that look organic and natural. To do this I created yet another grid of Perlin Noise values, mapping the rows and columns of the canvas window used in the Poisson Disk Sampling algorithm to new Perlin Noise values. Tree types are then determined by their Perlin Noise value in their row and column. This creates the effect of having bunches of pine trees, bunches of apple trees, and bunches of oak trees instead of a totally random assortment of the trees.
I wanted to make the project interactive, so I added a menu of settings that the user can use to create new forests. I made this menu with a very easy to use Javascript library called "dat.gui". Most of these menu options were straight forward to implement (like the zoom, tree density, and noise values, which are just variables that the user can adjust). The options to include or exclude certain trees, grass, and water took some work though. I had to create several conditional statements that adjusted certain probabilities and ratios based on what the user had selected as options for the new forest. If I were to keep working on this project, I would like to add a way for the user to adjust ratios of the various elements, but I'm satisfied with the project for now.
Overall, I am very happy with this project. It is fun to watch a little world get created by setting some variables and running some algorithms. I learned a lot about Perlin Noise and the Poisson Disk Sampling algorithm by trying to implement them together to create the illusion of a 2D forest. This project was full of fun little challenges and it has made me much more curious about computer graphics. I definitely want to explore more algorithms like these to see what I can make in the future.